JavaScript
The flexible language
JavaScript is Butter in Your Hands
Both Sides
Client and Server
Interpreters
The easy approach
How Do Interpreters Work?
- Read some source code
- Build an AST (Abstract Syntax Tree) by parsing the source code
- Evaluate the program by walking over the AST and performing instructions
Compilers for free
## AST
```js
x = 2; y = x * 3
```
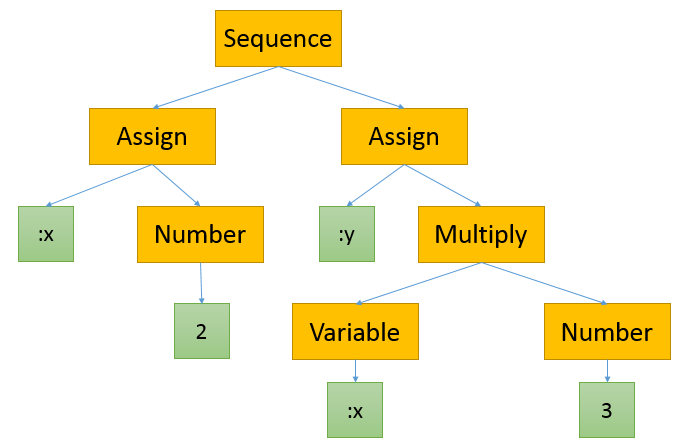
Compilers
Programming languages expressed in JavaScript
How Do Compilers Work?
- Read some source code
- Build an AST by parsing the source code
- Generate target code by walking the AST and emitting instructions
Compilers for free
## Expressions
```js
function DottedExpression(left, right) {
this.compile = function () {
return left.compile() + '.' + right.compile();
}
}
function BinaryExpression(oper, left, right) {
this.compile = function () {
return left.compile() + ' ' + oper + ' ' + right.compile();
}
}
```
## Commands
```js
function AssertCommand(expr) {
this.compile = function (options) {
var code = "if (__debug__ && !(" + expr.compile(options) + ")) { throw 'assert error' }";
return code;
}
}
function RaiseCommand(expr) {
this.compile = function (options) {
var code = "throw " + expr.compile(options) + ";";
return code;
}
}
```
## TDD as usual ;-)
```js
exports['Compile return command'] = function (test) {
test.equal(compileCommand(test, 'return 1'), 'return 1;');
}
exports['Compile call expressions'] = function (test) {
test.equal(compileExpression(test, 'print()'), 'print()');
test.equal(compileExpression(test, 'print(1)'), 'print(1)');
}
exports['Compile call command'] = function (test) {
test.equal(compileCommand(test, 'print()'), 'print();');
test.equal(compileCommand(test, 'print(1)'), 'print(1);');
}
```
## Virtual Machine, Bytecodes
```js
x = 2; y = x * 3
```
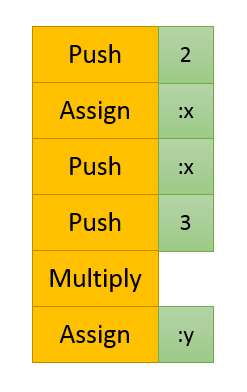
The End
BY Angel 'Java' Lopez / www.ajlopez.com / @ajlopez